Understanding LangChain: A Comprehensive Guide
Discover LangChain, the innovative framework simplifying app development with language models (LLMs). Learn about its core components—chains, agents, tools, memory, and callbacks—and how they enable dynamic, context-aware applications. Ready to harness the power of LLMs? Dive into LangChain and e...
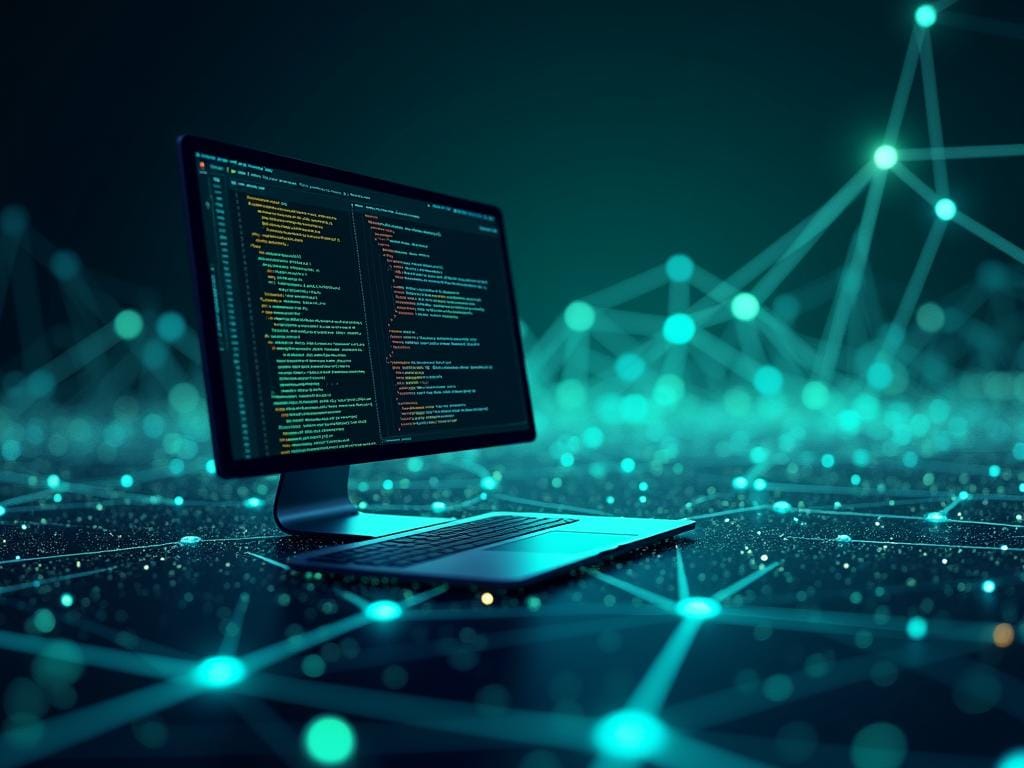
LangChain is an innovative framework designed to facilitate the development of applications that leverage language models (LLMs). It provides a structured way to build applications that can interact with various data sources, perform complex reasoning, and generate human-like text. In this blog post, we will explore how LangChain works, its core components, and how you can utilize it to create powerful applications.
What is LangChain?
LangChain is a framework that allows developers to create applications powered by language models. It provides a set of tools and abstractions that make it easier to work with LLMs, enabling developers to build applications that can understand and generate natural language. The framework is designed to be modular, allowing developers to mix and match components to suit their specific needs.
Key Features of LangChain
- Modularity: LangChain is built with modularity in mind. Developers can choose from various components, such as chains, agents, and tools, to create custom workflows.
- Integration with LLMs: LangChain seamlessly integrates with popular language models, allowing developers to leverage their capabilities without dealing with the complexities of model management.
- Data Handling: The framework provides tools for handling different types of data, including text, structured data, and external APIs.
- Evaluation and Testing: LangChain includes features for evaluating the performance of language models and testing the outputs of applications.
- Community and Documentation: LangChain has a growing community and extensive documentation, making it easier for developers to get started and find support.
Core Components of LangChain
LangChain consists of several core components that work together to create powerful applications. Here are some of the key components:
1. Chains
Chains are the building blocks of LangChain applications. A chain is a sequence of operations that process input data and produce output. Chains can be simple or complex, depending on the application's requirements.
- Example: A simple chain might take a user query, pass it to a language model, and return the generated response. A more complex chain could involve multiple steps, such as data retrieval, processing, and summarization.
2. Agents
Agents are intelligent components that use language models to determine a sequence of actions to take based on user input. Unlike chains, which have a predefined sequence of operations, agents can dynamically decide which actions to perform.
- Example: An agent could analyze a user's question and decide whether to retrieve information from a database, generate a response using a language model, or perform a calculation.
3. Tools
Tools are external functions or APIs that agents can use to perform specific tasks. LangChain provides a variety of built-in tools, and developers can also create custom tools to extend functionality.
- Example: A tool could be a function that retrieves data from an external API, performs a calculation, or processes text.
4. Memory
Memory is a crucial component that allows LangChain applications to maintain state across interactions. It enables agents to remember previous inputs and outputs, providing a more coherent and context-aware user experience.
- Example: A chatbot built with LangChain could use memory to remember the user's name and preferences, allowing for personalized interactions.
5. Callbacks
Callbacks are functions that can be executed at various points during the execution of a chain or agent. They allow developers to track the progress of operations, log information, or handle errors.
- Example: A callback could log the inputs and outputs of a chain for debugging purposes.
How Does LangChain Work?
LangChain operates by combining its core components to create a cohesive workflow. Here's a high-level overview of how LangChain applications typically work:
- Input Handling: The application receives input from the user, which could be a question, command, or data.
- Routing: If an agent is used, it analyzes the input and determines the appropriate actions to take. This may involve selecting a specific chain or tool based on the input.
- Processing: The selected chain or tool processes the input. This could involve querying a database, generating text with a language model, or performing calculations.
- Output Generation: The processed output is generated and returned to the user. If memory is used, the application may also store relevant information for future interactions.
- Evaluation: The output can be evaluated against predefined criteria to assess its quality and relevance. This is particularly useful for applications that require high accuracy.
Getting Started with LangChain
To get started with LangChain, you'll need to install the framework and set up your development environment. Here's a step-by-step guide:
Step 1: Install LangChain
You can install LangChain using pip. Open your terminal and run the following command:
pip install langchain
Step 2: Set Up Your Language Model
LangChain supports various language models, including OpenAI's GPT-3 and GPT-4. You'll need to set up an API key for the model you choose to use.
Step 3: Create Your First Chain
Here's a simple example of creating a chain that generates a response based on user input:
from langchain.chains import LLMChain
from langchain.llms import OpenAI
# Initialize the language model
llm = OpenAI(api_key="your_api_key")
# Create a simple chain
chain = LLMChain(llm=llm)
# Run the chain with user input
response = chain.run("What is the capital of France?")
print(response)
Step 4: Explore More Features
Once you have a basic chain set up, you can explore more advanced features, such as creating agents, using memory, and integrating tools. The LangChain documentation provides comprehensive guides and examples to help you along the way.
Useful Links and Resources
- LangChain Documentation
- LangChain GitHub Repository
- LangChain Hub
- LangChain Examples
- LangChain Community
Conclusion
LangChain is a powerful framework that simplifies the development of applications powered by language models. Its modular design, integration with various data sources, and support for dynamic decision-making make it an excellent choice for building intelligent applications. By understanding its core components and how they work together, you can leverage LangChain to create innovative solutions that harness the power of language models.
Whether you're building a chatbot, a data analysis tool, or a content generation application, LangChain provides the tools and flexibility you need to succeed. Start exploring LangChain today and unlock the potential of language models in your projects!
Comments ()