Automatically Flag Apple Emails with Ollama AI (Source)
This blog post delves into an AppleScript designed to automate email categorization through a user-defined logging system. It processes email messages, analyzes their content, and categorizes them based on predefined color flags. The script effectively captures log details, managing errors and en...
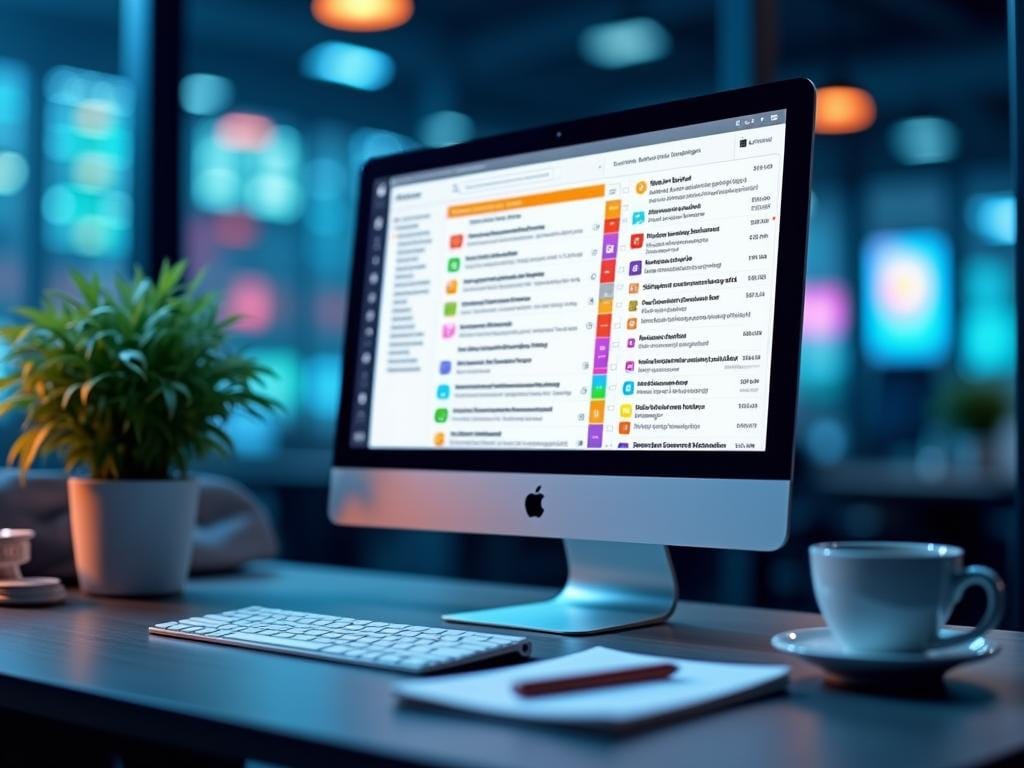
on appendLog(logMessage)
try
set homePath to (do shell script "echo $HOME")
set logFile to homePath & "/Library/Logs/ollama-mail-flags.log"
set timeStr to do shell script "date '+%Y-%m-%d %H:%M:%S'"
set logLine to timeStr & " - " & logMessage
do shell script "printf '%s\\n' " & quoted form of logLine & " >> " & quoted form of logFile
on error errStr
display dialog "Logging Error: " & errStr
end try
end appendLog
using terms from application "Mail"
on perform mail action with messages messageList
-- List mapping colors to their flag numbers (color, flag number)
set colorFlags to {{"Red", 0}, {"Orange", 1}, {"Yellow", 2}, {"Green", 3}, {"Blue", 4}, {"Purple", 5}, {"Grey", 6}}
-- List mapping colors to categories (color, category)
set colorCategories to { {"Blue", "News"}, {"Purple","Invoice"}, {"Grey","Work"}}
-- Initialize log file
my appendLog("=== Starting new mail processing session ===")
-- Log available categories
set catStr to ""
repeat with i from 1 to count of colorCategories
set colorCat to item i of colorCategories
set theColor to item 1 of colorCat
set theCategory to item 2 of colorCat
set catStr to catStr & theCategory & " (" & theColor & "), "
end repeat
set catStr to catStr & "Other"
my appendLog("Available categories: " & catStr)
tell application "Mail"
try
repeat with theMessage in messageList
set msgContent to ""
try
set msgContent to content of theMessage as text
set msgSubject to subject of theMessage
my appendLog("Processing message: " & msgSubject)
on error
-- Skip this message if content can't be retrieved
my appendLog("Error: Could not retrieve message content")
cycle
end try
-- Check message content before processing
if msgContent is "" then
my appendLog("Error: Empty message content")
cycle
end if
-- Truncate content to 100 words
set wordCount to 0
set truncatedContent to ""
set theWords to every word of msgContent
repeat with i from 1 to count of theWords
if wordCount ≥ 100 then
exit repeat
end if
set truncatedContent to truncatedContent & " " & (item i of theWords)
set wordCount to wordCount + 1
end repeat
if wordCount ≥ 100 then
set truncatedContent to truncatedContent & "..."
end if
my appendLog("Content: " & truncatedContent)
-- Remove all special whitespace characters and escape
set escapedContent to do shell script "printf %s " & quoted form of truncatedContent & " | tr -d '\n\t\r' | sed 's/[\"\\]/\\&/g'"
-- Build category options string
set categoryOptions to ""
repeat with i from 1 to count of colorCategories
set colorCat to item i of colorCategories
set theColor to item 1 of colorCat
set theCategory to item 2 of colorCat
set categoryOptions to categoryOptions & "- " & theCategory & " (" & theColor & ")" & " "
end repeat
set categoryOptions to categoryOptions & "- Other"
set thePrompt to "Based on the following text, select the most appropriate category from the options below. Answer with ONLY the category name (Critical, News) or Other, no explanation: Text: " & escapedContent & " Options: " & categoryOptions
my appendLog("Prompt sent to Ollama: " & thePrompt)
set jsonData to "{\"model\": \"llama3.1:latest\", \"prompt\": \"" & thePrompt & "\"}"
set curlCommand to "curl http://localhost:11434/api/generate -d " & quoted form of jsonData
try
set response to do shell script curlCommand
my appendLog("Raw response: " & response)
-- Find category's color
repeat with i from 1 to count of colorCategories
set colorCat to item i of colorCategories
set theColor to item 1 of colorCat
set theCategory to item 2 of colorCat
if response contains theCategory then
-- Find color's flag number
repeat with j from 1 to count of colorFlags
set colorFlag to item j of colorFlags
set flagColor to item 1 of colorFlag
set flagNumber to item 2 of colorFlag
if flagColor is equal to theColor then
set flag index of theMessage to flagNumber
my appendLog("Flag set: " & theCategory & " (" & theColor & ")")
exit repeat
end if
end repeat
exit repeat
end if
end repeat
on error errStr
my appendLog("API Error: " & errStr)
display dialog "Curl Error: " & errStr
end try
end repeat
my appendLog("=== Mail processing session completed ===")
return true
on error errStr
my appendLog("Script Error: " & errStr)
display dialog "Error: " & errStr
return false
end try
end tell
end perform mail action with messages
end using terms from