Architecture-driven code generation with Claude Dev and Gpt-4
Discover a transformative coding strategy with architecture-driven code generation! By using LLMs like Claude to generate architecture documentation before diving into coding, you enhance code maintainability and clarity. This post guides you through creating a mind map app, showcasing the benefi...
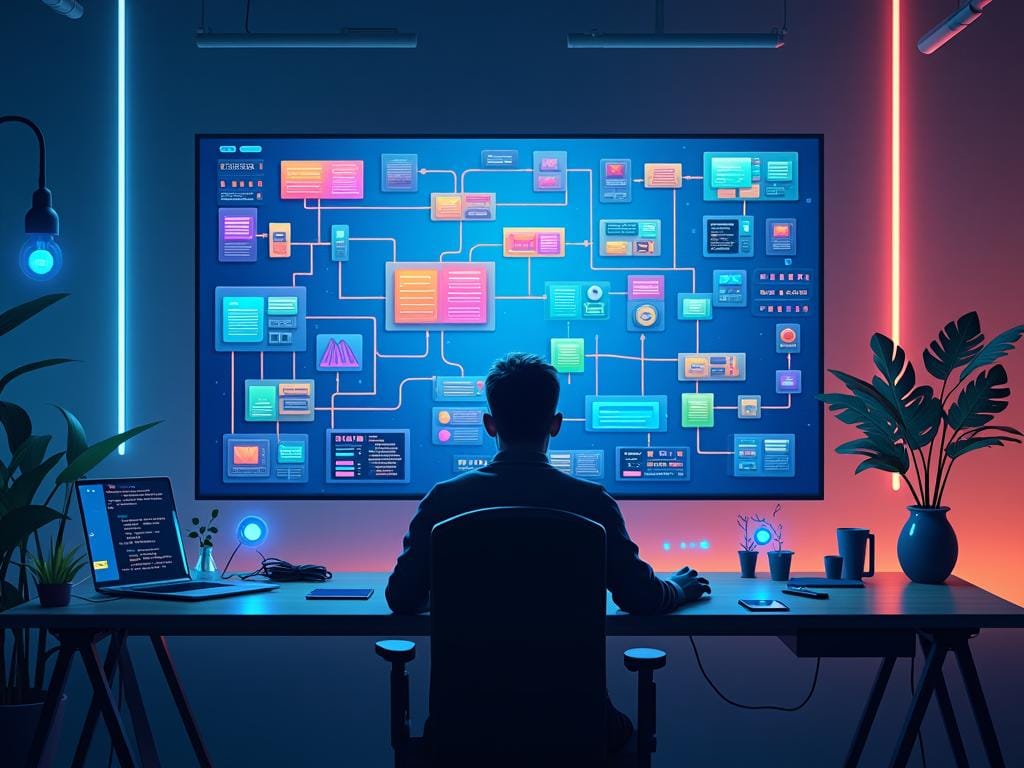
I've Been using ChatGPT and Claude to generate code for a while now, and one of the challenges we face with LLMs in code generation is the trade-off between ease and maintainability. The easier it becomes to create code, the more challenging it will be for humans to understand every detail, and the pressure to ship new features quickly will put a strain on code quality.
The easier it becomes to create code, the more challenging it will be for humans to understand every detail, and the pressure to ship new features quickly will put a strain on code quality.
With this in mind, I started using a different approach. I use the LLM to first generate architecture documentation and diagrams to solve the problem, and THEN start coding. This has many benefits:
- It makes the LLM think about a clean structure and smooth integration of various parts of the code before actually coding.
- It helps keep an overall understanding of the main components of the application as it grows.
- The documentation (not just code documentation but also sequence diagrams, class diagrams, etc.) reflects the real code and stays in sync.
- It eventually makes the code much more maintainable.
I call this approach architecture-driven code generation.
In this post, we'll explore this novel approach for architecture-driven code generation.
To demonstrate the idea, I'll take you through a short tutorial on creating a mind map using the architecture-first approach, and adding features incrementally using the same approach. In the end, we should end up with something like this:
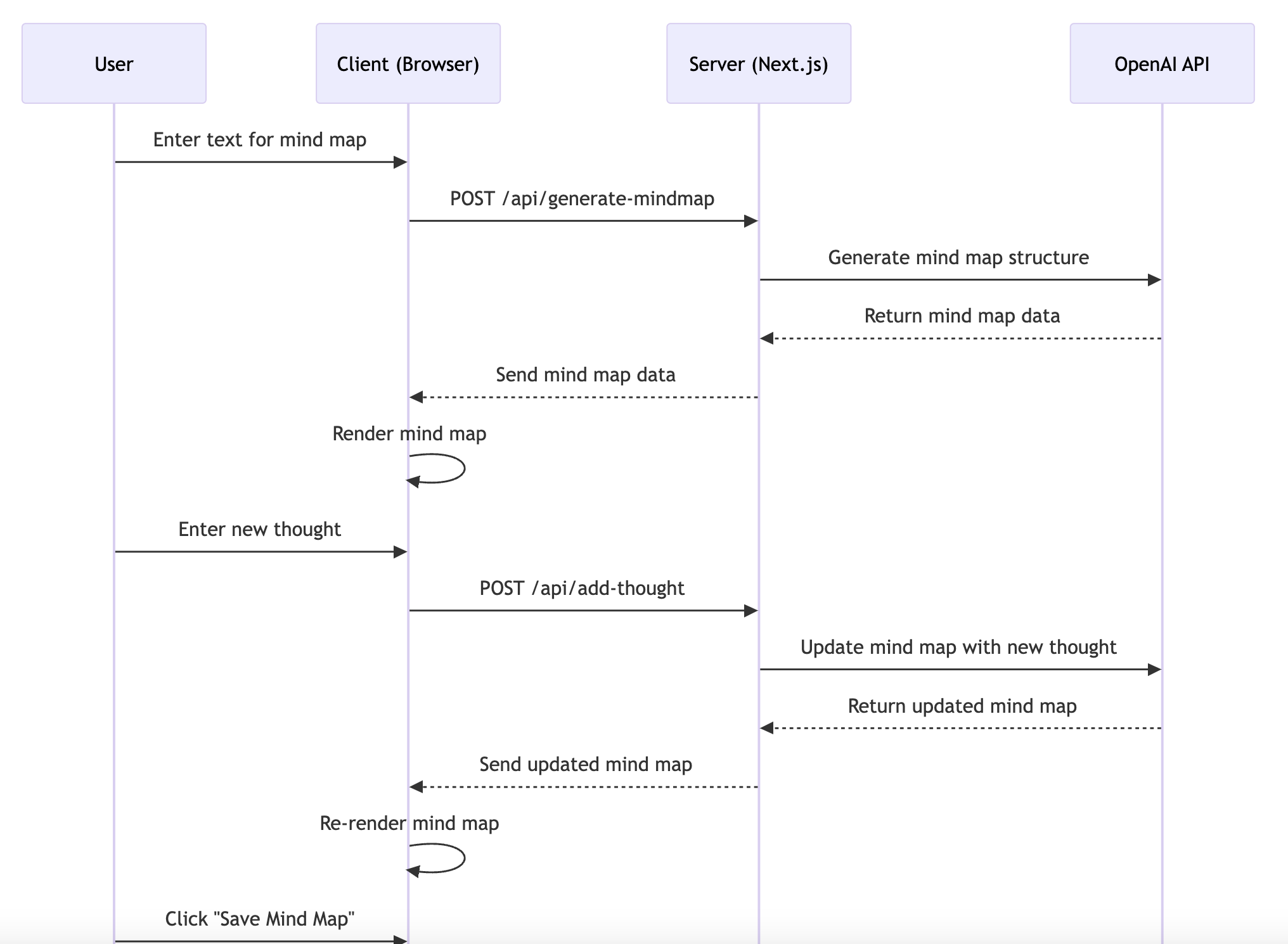
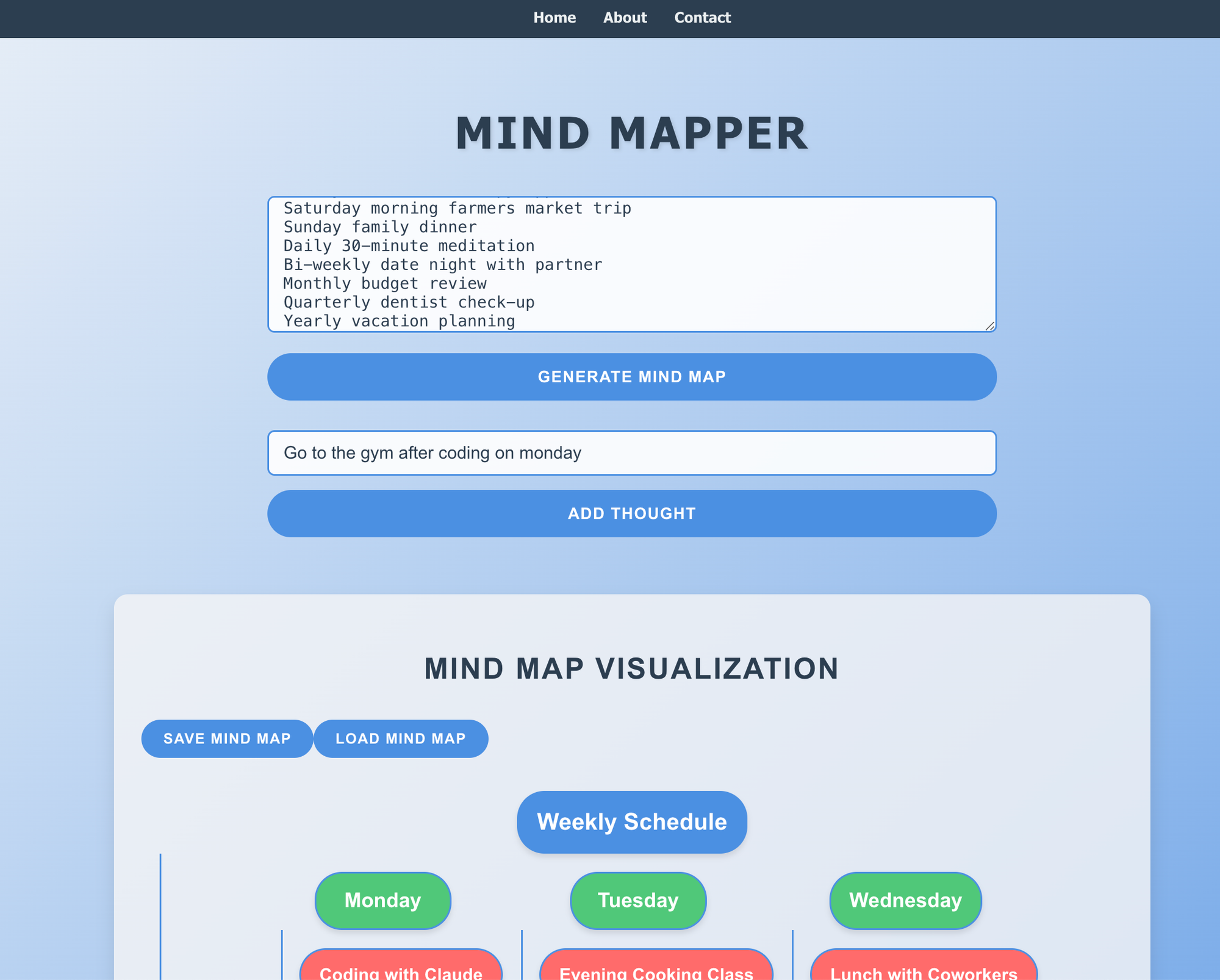
For this tutorial, I chose Claude because, in my opinion, it's the best code generator out there. And with the Claude Dev plugin in VSCode, incremental updates to the code are much easier than using Copilot or copy-pasting between the chat and my codebase. The Claude Dev plugin can be downloaded here. Of course, you can use any other suitable plugin if you wish.
Please note that during this tutorial, you might get different results because LLMs can be unpredictable, but the overall strategy should work with any LLM that's well-trained for code generation.
So let's start...
The functional requirements are very simple:
"I want to be able to create a mind map from natural language and be able to update the mind map with updates that are automatically fed into the same mind map." Finally, we should be able to save and load the mind map to do incremental updates to it. That's it.
Here is a sample input to start with:
Monday morning gym session
Tuesday evening cooking class
Wednesday lunch with coworkers
Thursday night book club meeting
Friday afternoon therapy appointment
Saturday morning farmers market trip
Sunday family dinner
Daily 30-minute meditation
Bi-weekly date night with partner
Monthly budget review
Quarterly dentist check-up
Yearly vacation planning
Step: Configure the Claude Dev Plugin:
Make sure to set auto-read; otherwise, you will have to confirm a lot.
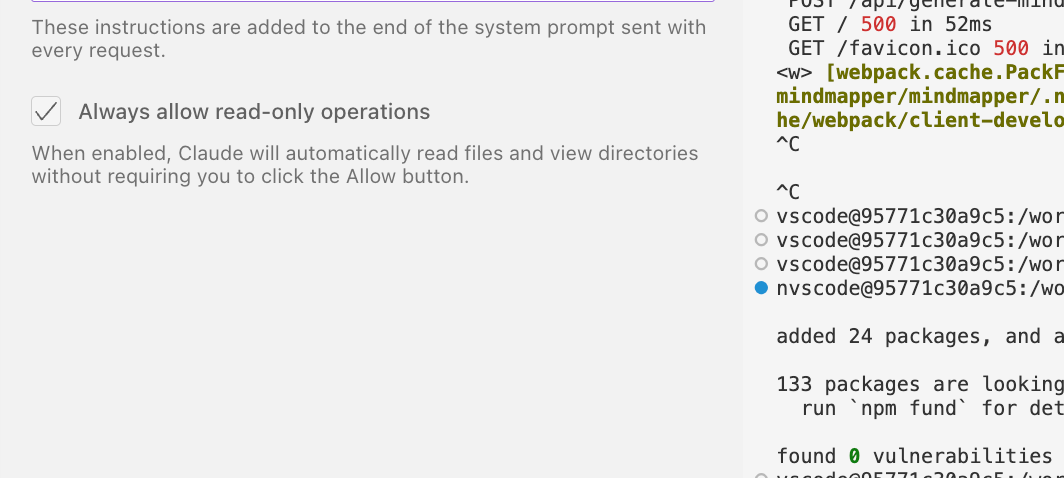
Step: Prompt Claude to generate the architecture diagrams
You are a helpful developer and architect.
Use Mermaid to create a sequence and architecture diagram for an application with the following requirements:
The user enters some text,
You call OpenAI in the background and ask it to create a Mermaid mind map.
You display this mind map in the UI.
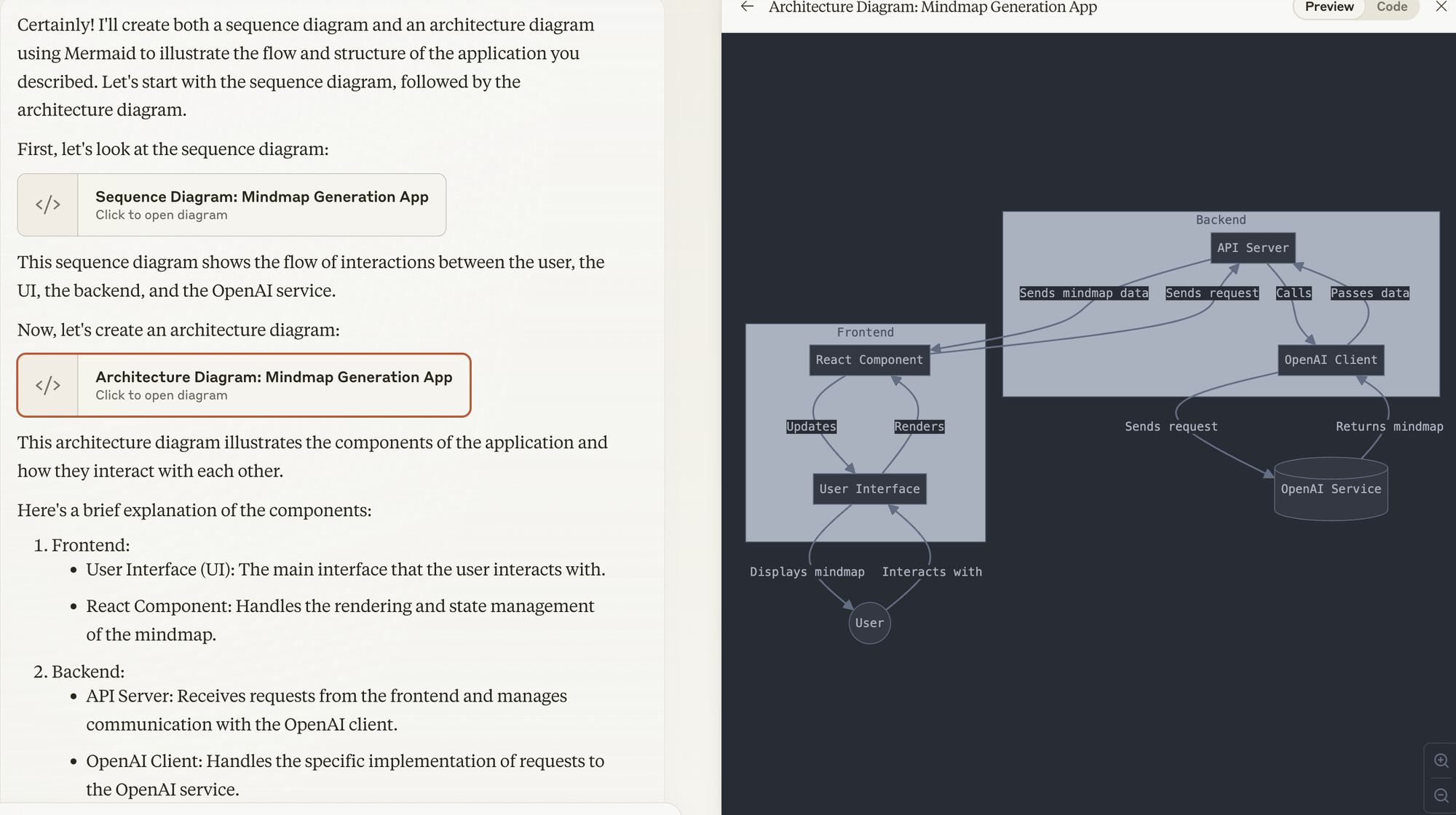
Then I asked Claude explicitly to use Next.js.
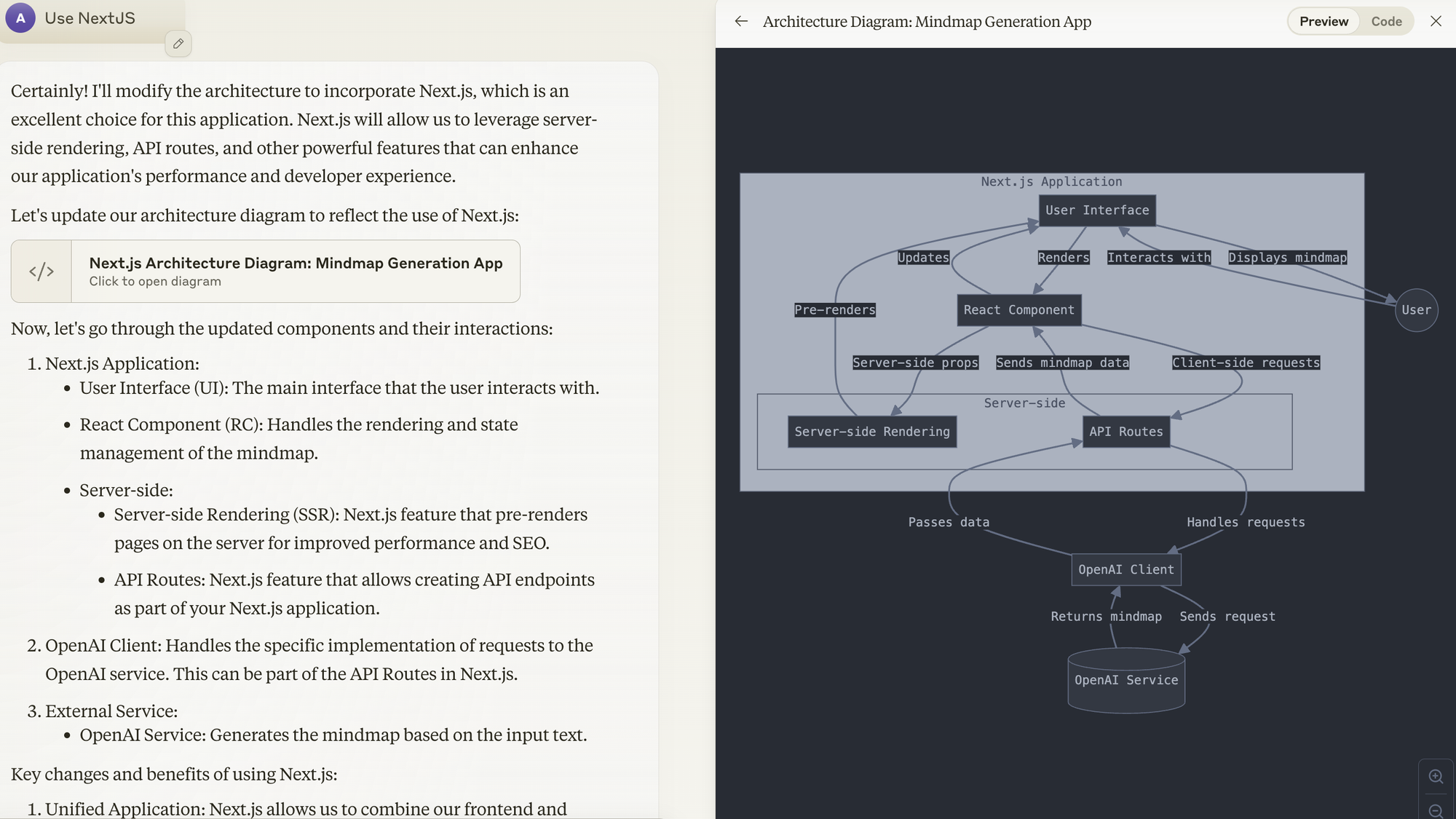
If everything looks good, we can go ahead and let Claude generate the code based on the architecture diagram. Note that as we are in the same chat session, it already knows "what to do."
Create Next.js Application based on the architecture diagram.
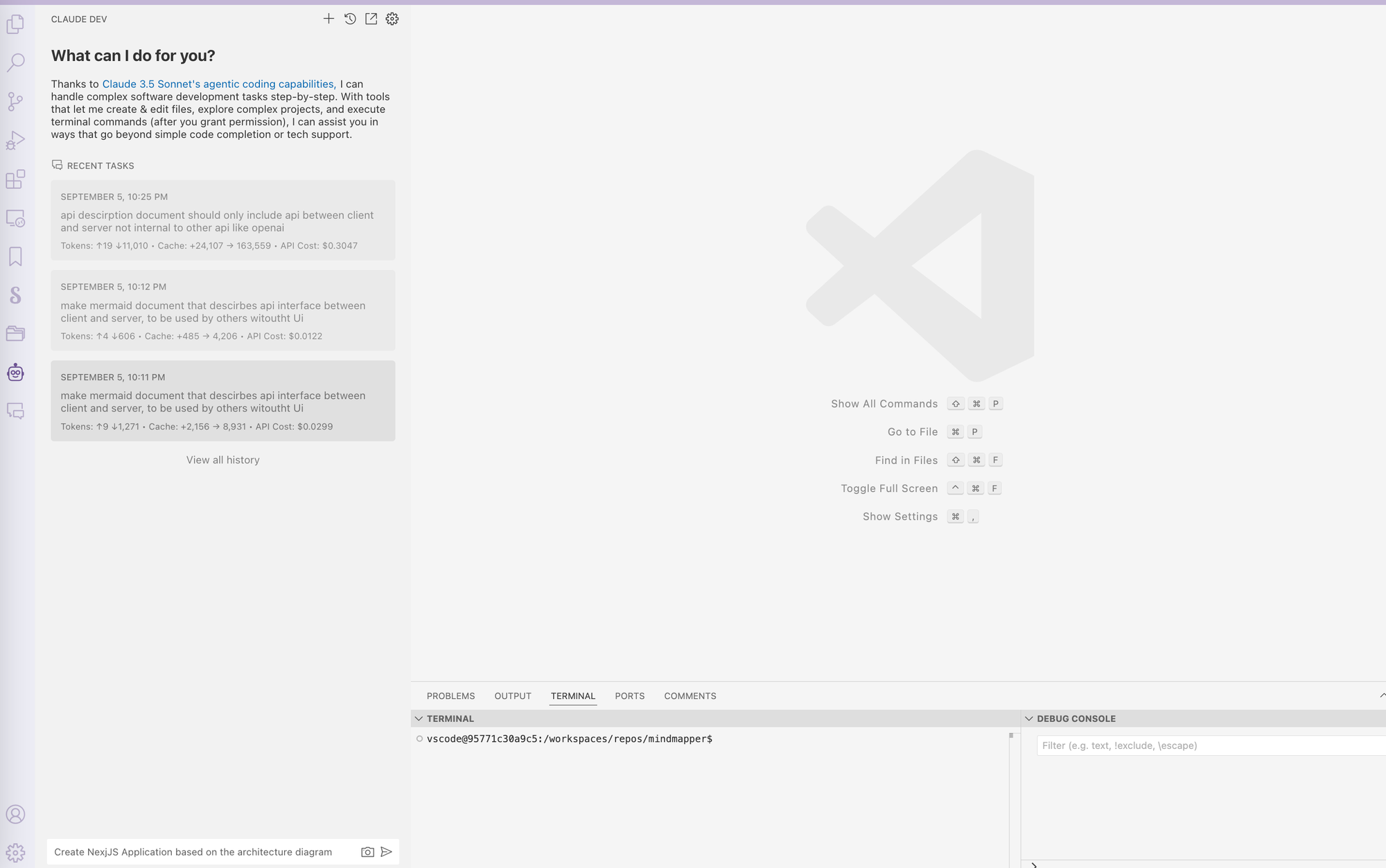
Claude Dev will read all the existing files and ask you to generate and edit files and execute code to create the Next.js application. Click Accept/Create/Save.
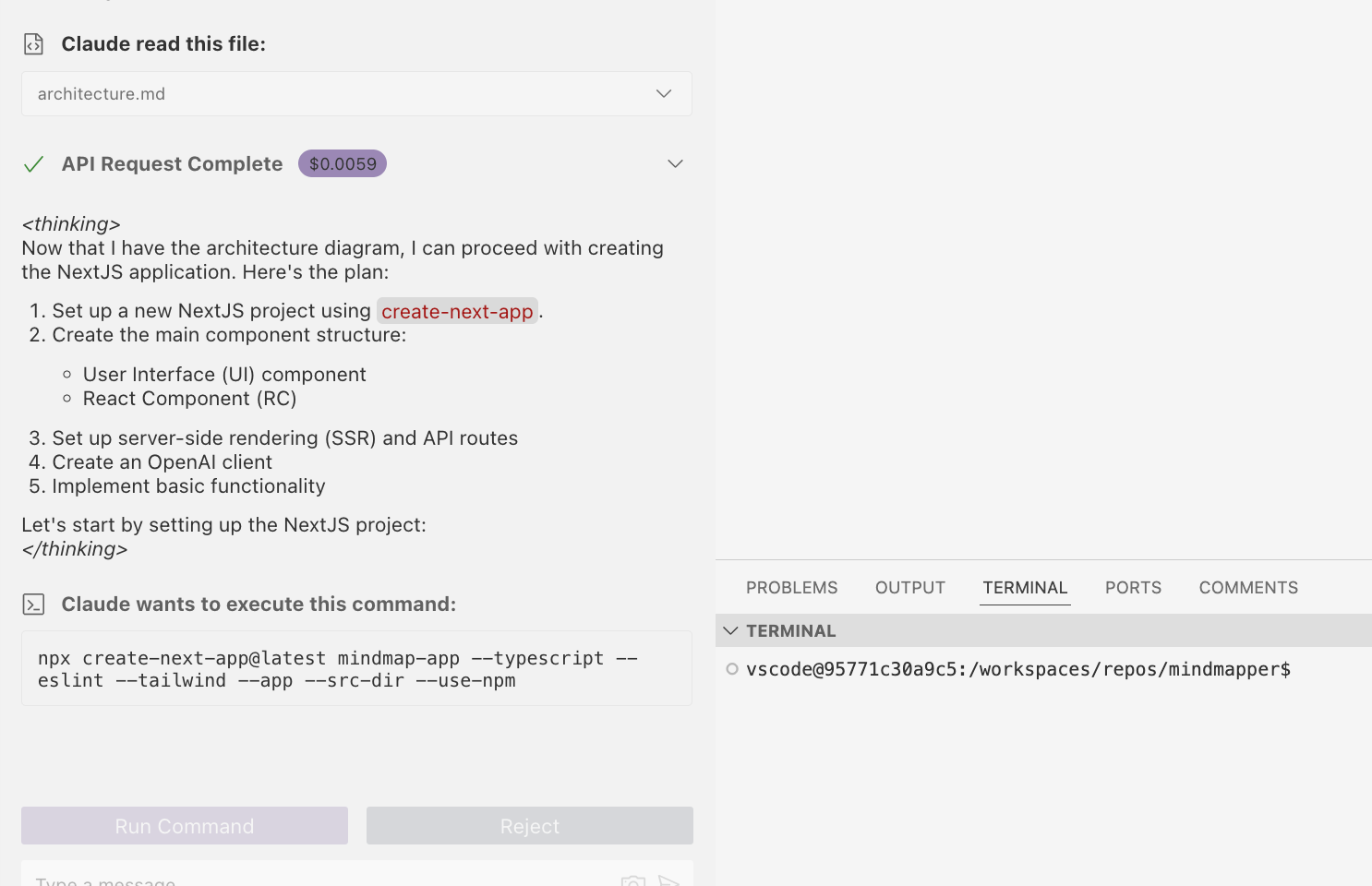
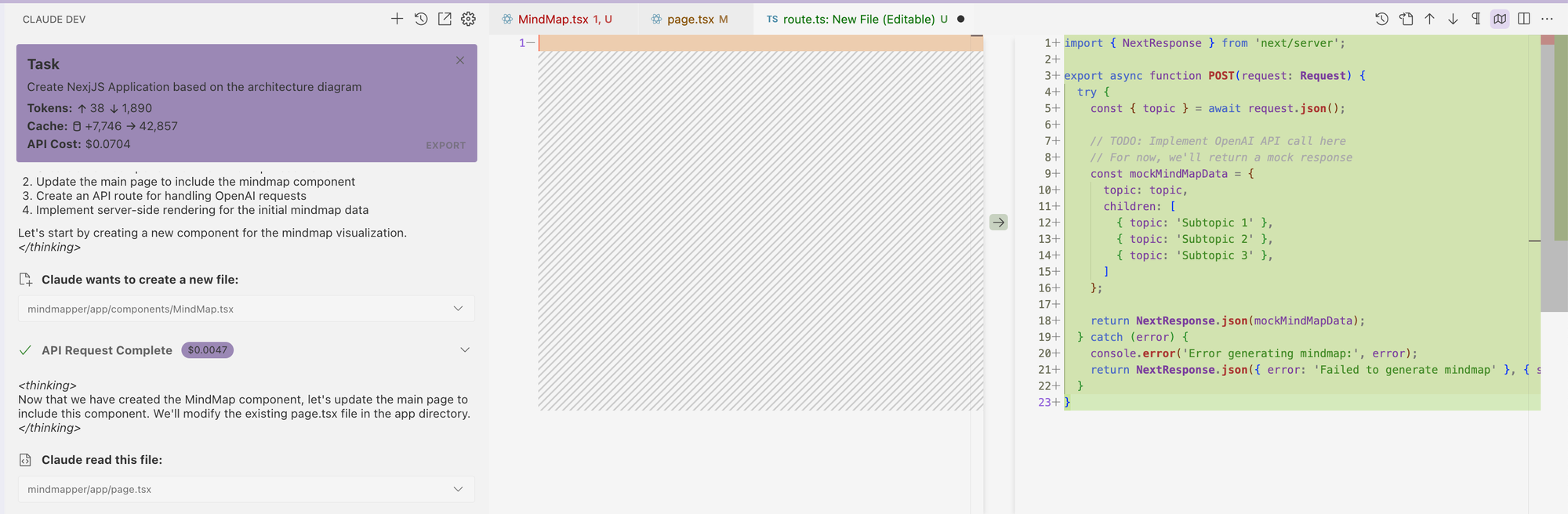
A few things to consider before actually starting the application
In some cases you may have to do some small manual work. Here are a few things I had to tweak before running the application.
Make sure to run npm install
before you start the application.
npm install
If OpenAI is not installed, make sure to install it.
npm install openai
And set your OpenAI API key.
export OPENAI_API_KEY=sk-xxxx
Make sure to replace gpt-3.5
in case it is used with gpt-4.0-mini
. The same in case Claude used any other outdated or expensive model not necessary for our use case, e.g., gpt-4
.
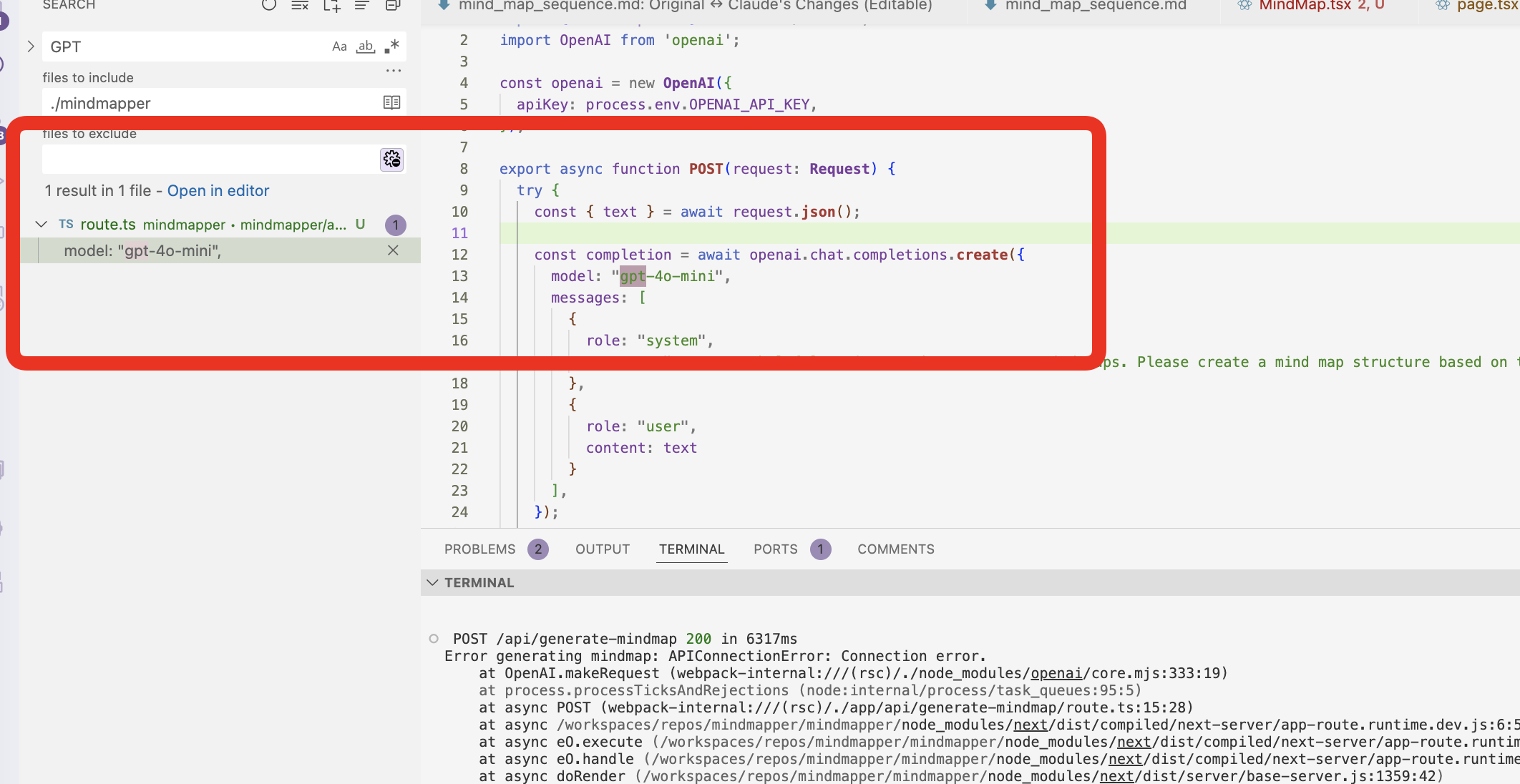
Then now you can start the app.
npm run dev
Now let's look at the result at localhost:3000
.
When all is said and done, you should be able to see something like this. The application should be a fully functional Next.js application in line with the agreed architecture.
But it looks a bit boring and not perfect yet, so let's tweak it a bit.
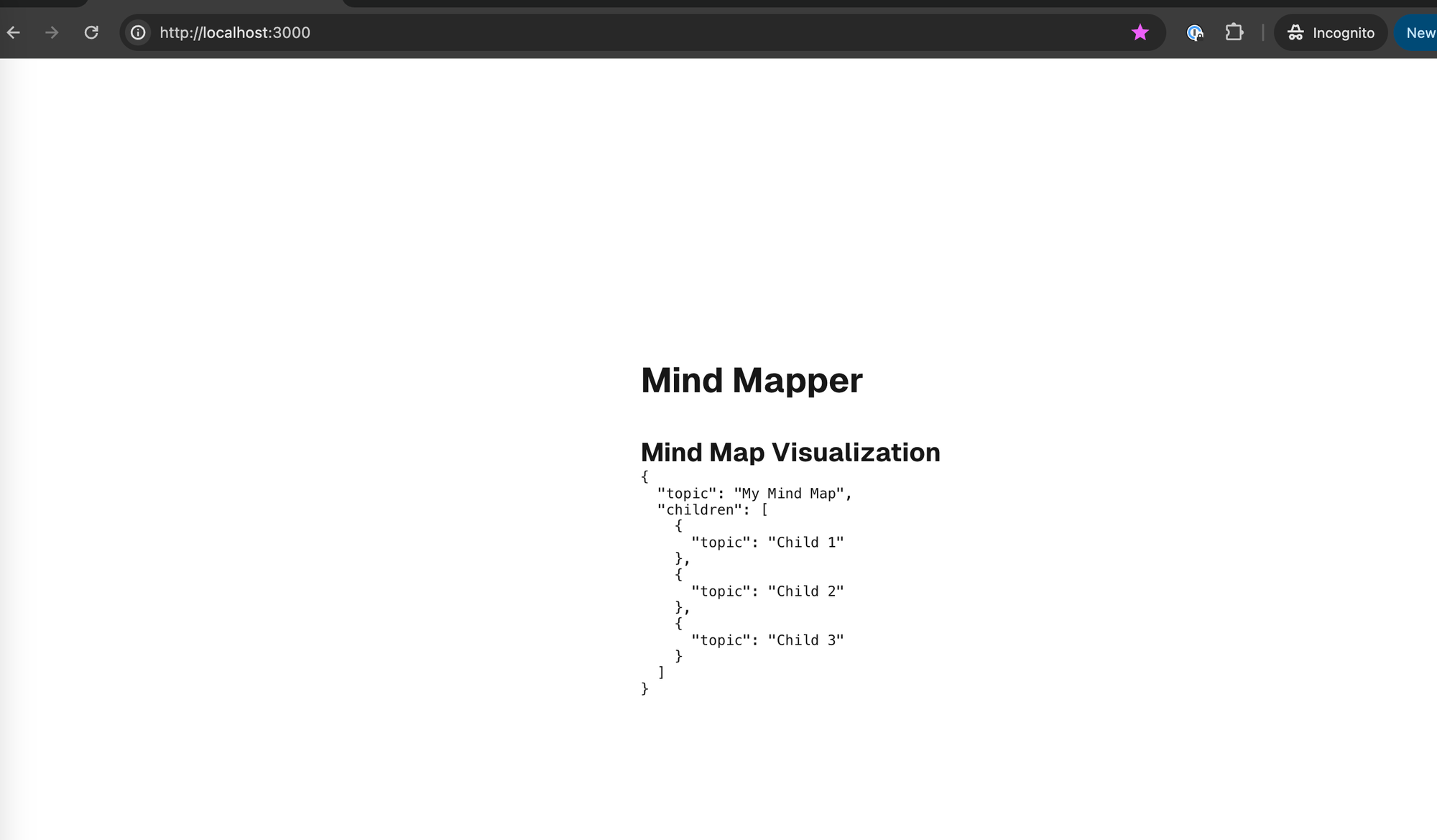
The user should be able to enter text, send it to OpenAI, and ask it to generate a Mermaid mind map, and then display it to the user: create a sequence diagram in Mermaid to demonstrate this behavior first. Do not code yet.
Again, let's update our sequence diagram first and make sure that the requirements in our head match Claude's understanding. In my case it did. If you get different unsatisfactory results, do a few iterations until it meets your expectations. Remember, this is the reference for the code generation, the contract between you and Claude.
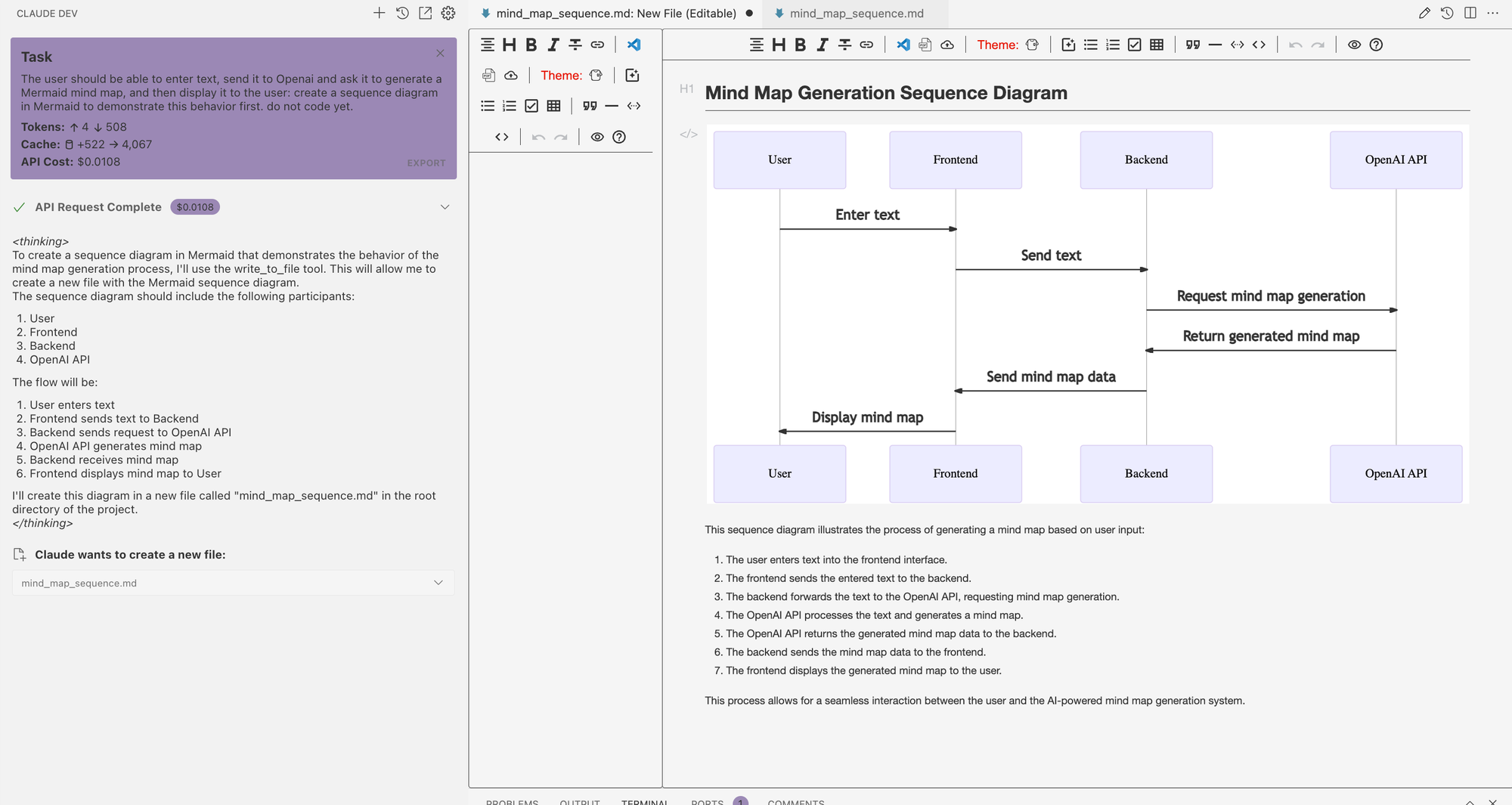
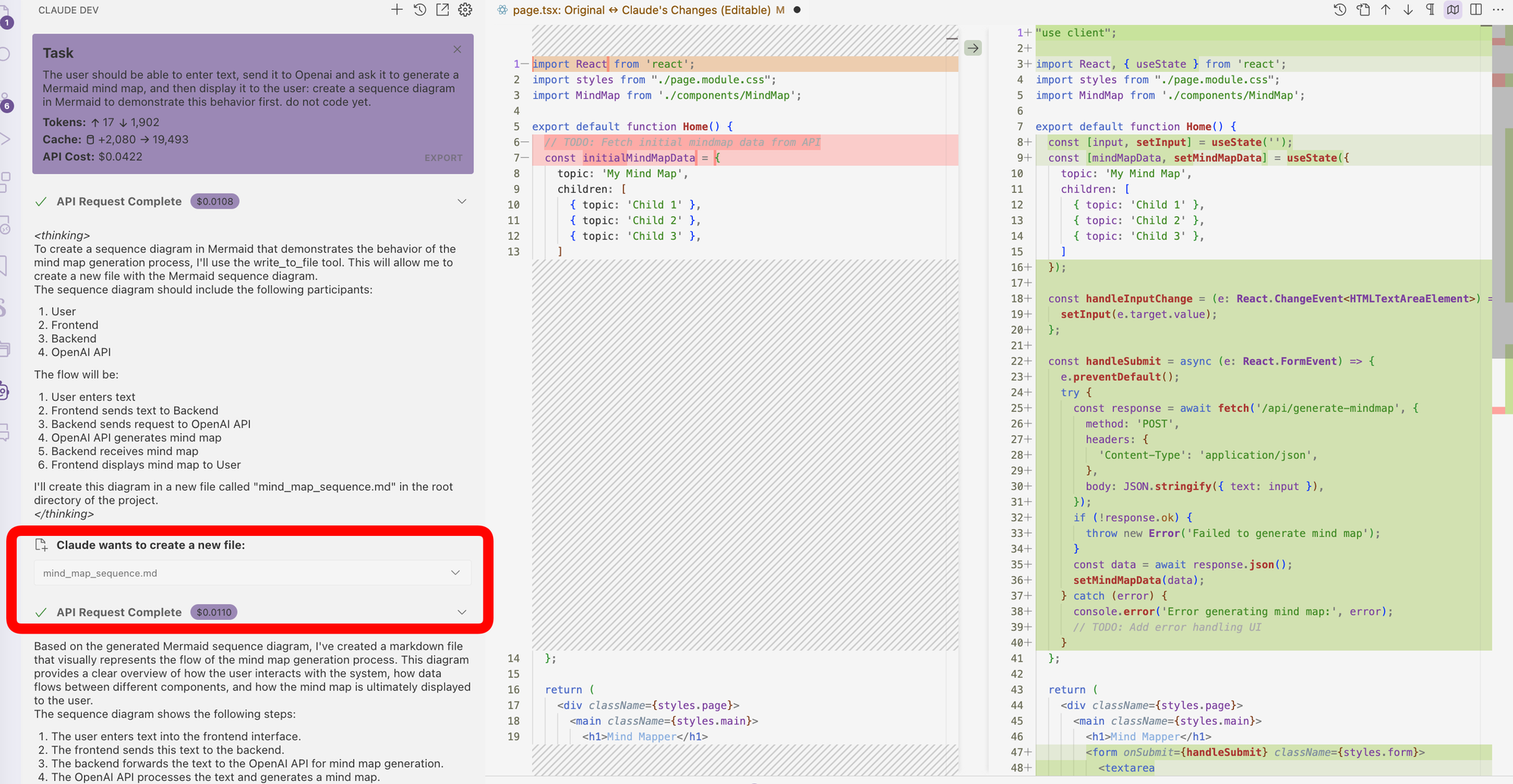
Now let's look again at localhost:3000
. It looks better.
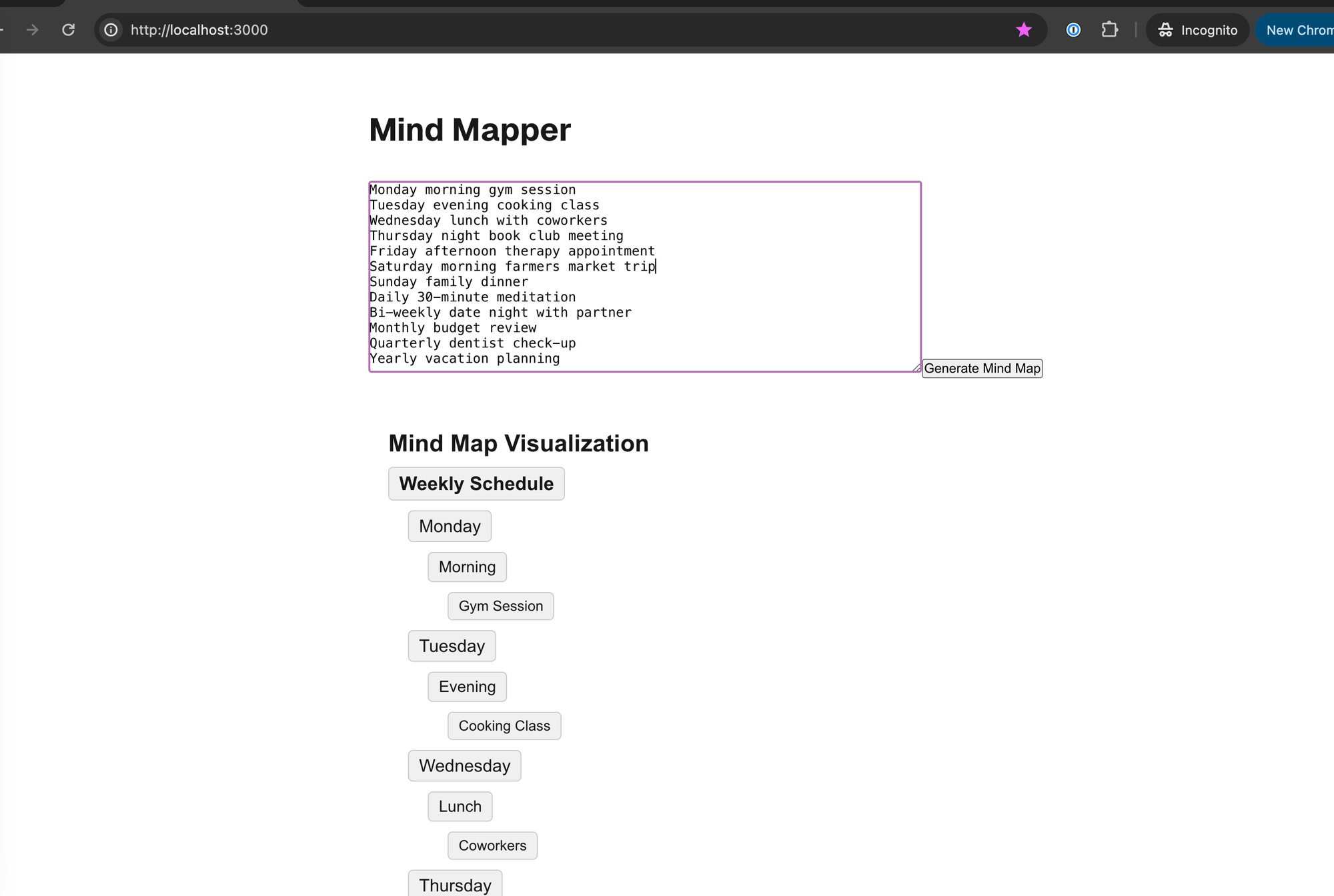
After a few more iterations, we can get something like this with just a few more iterations.
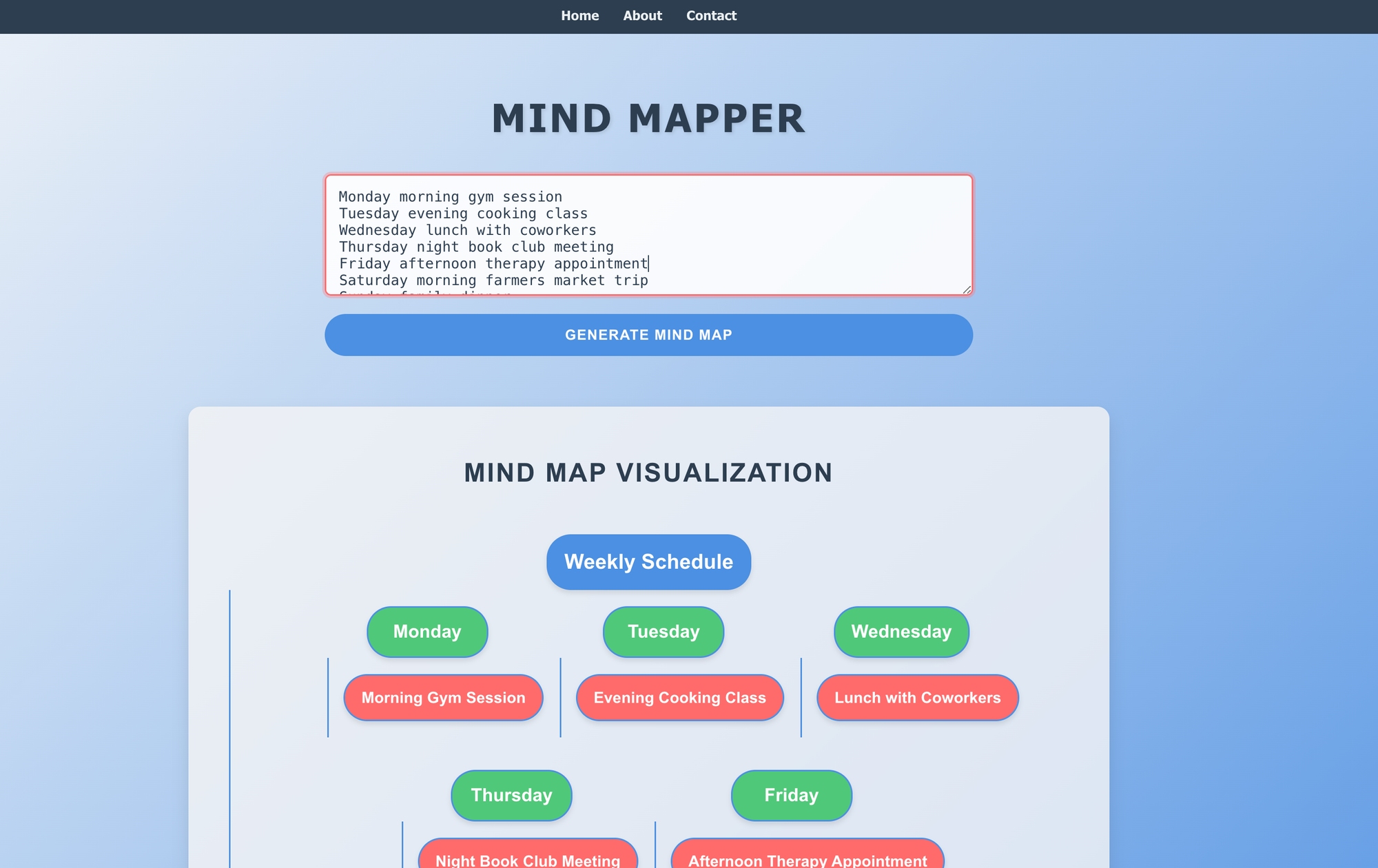
Now we want to add a new feature: to save the mind map. And again, we start with the architecture.
Update all diagrams to reflect a new feature:
The user should be able to save and load the file locally.
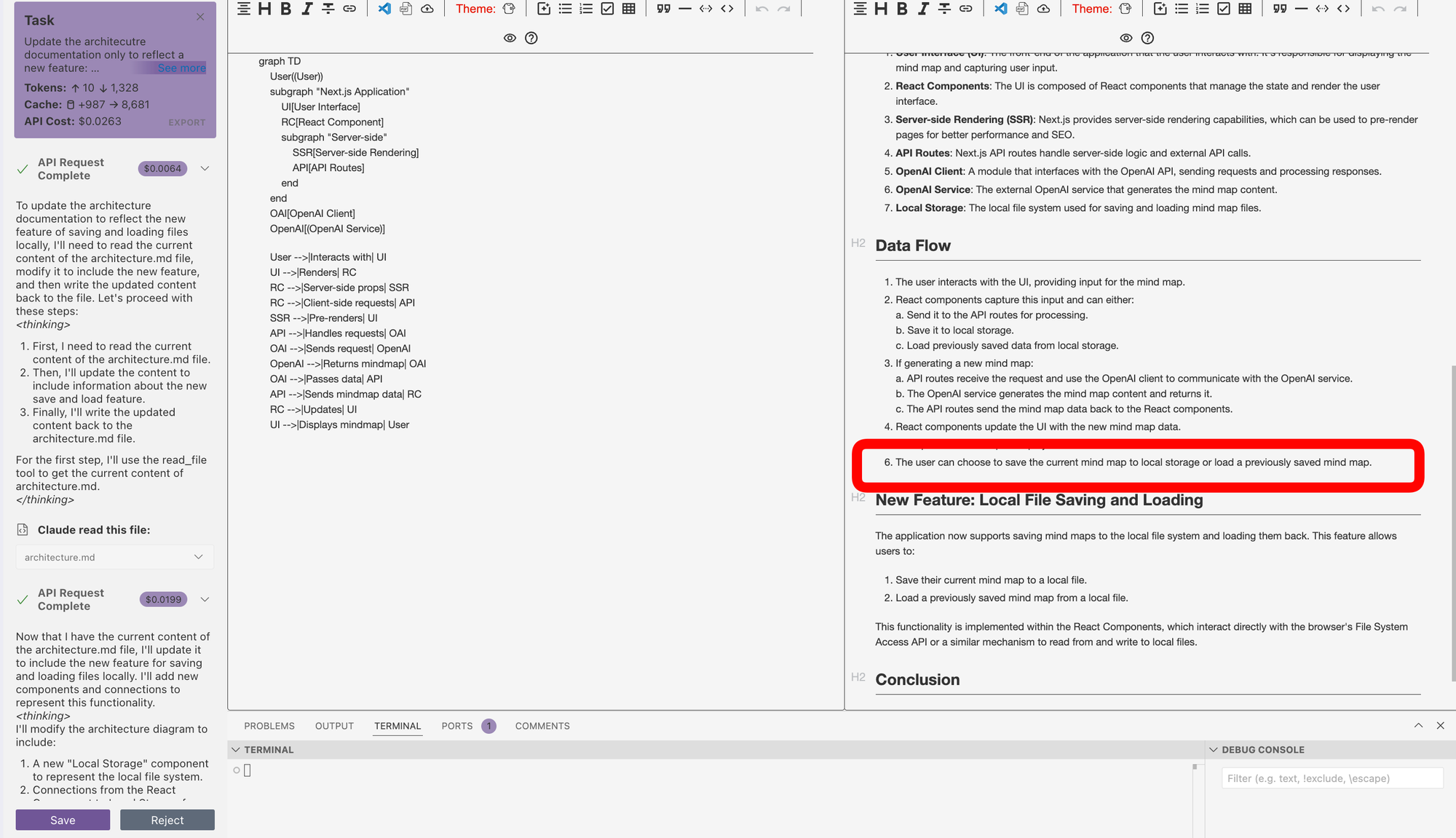
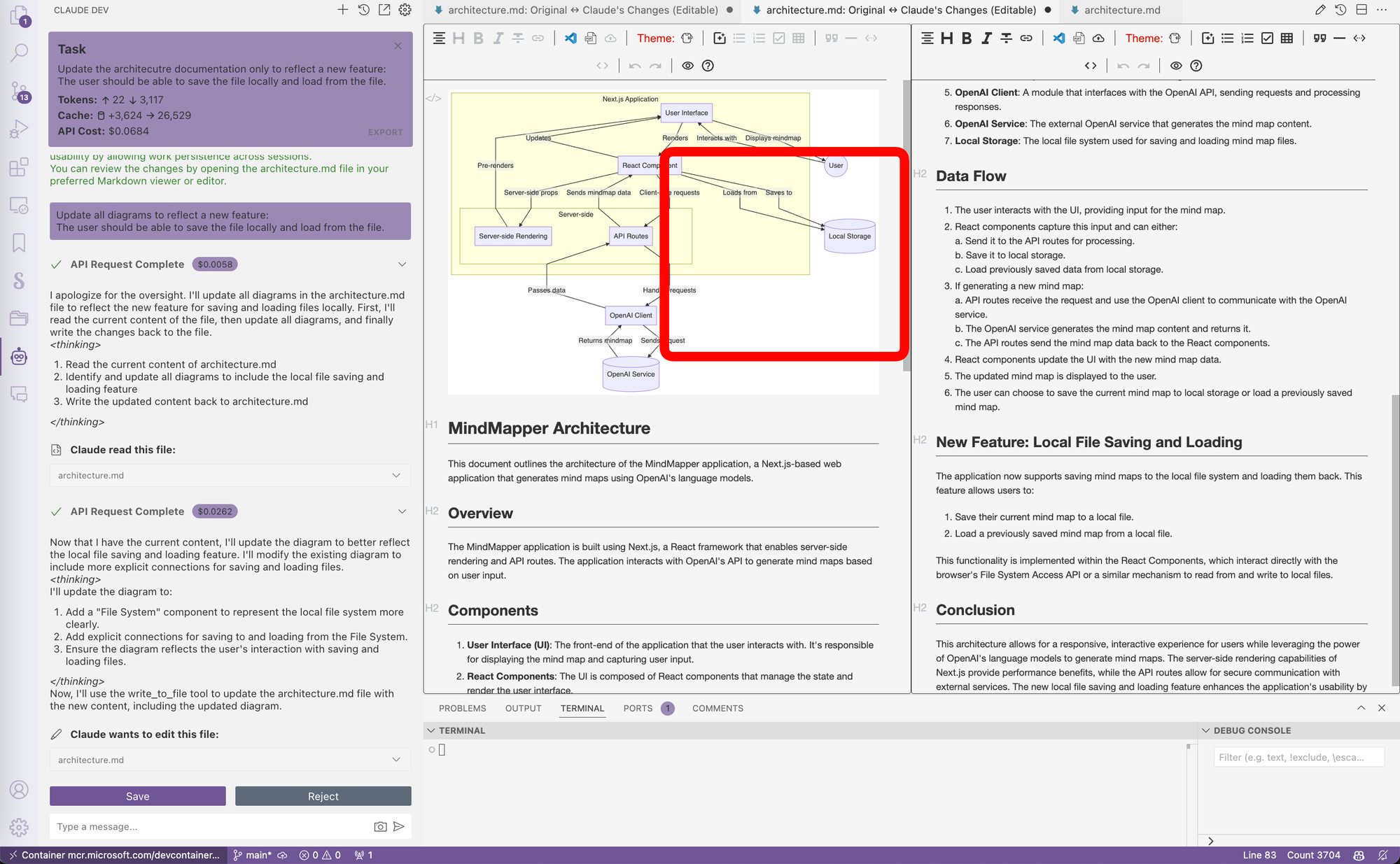
As you can see from the diagrams (sequence diagram and state chart), it has added the new feature of saving to local storage successfully. It looks good.
If it does not meet your expectations, this is the place to iterate and refine the architecture until you have a clear architecture to implement.
Now let's implement the new feature according to the documentation.
Make sure you stay in the same chat, so that Claude has some context about the new feature to be implemented.
Implement the new feature in the code.
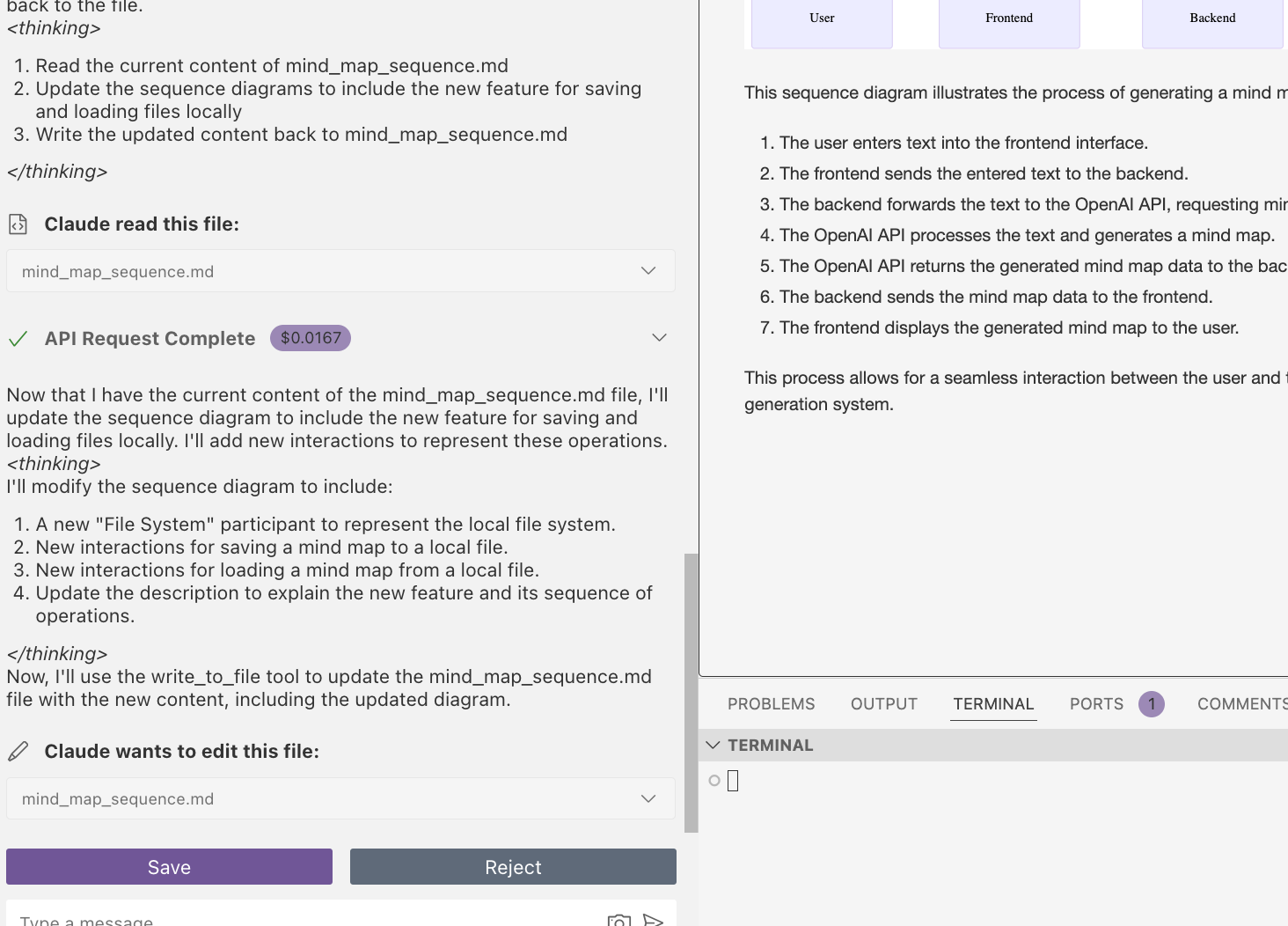
After all implementation is okay, we can now see the results.
I save the mind map as test.json and load and change the gym session on Monday to “Coding with Claude”.
{"topic":"Weekly Schedule","children":[{"topic":"Monday","children":[{"topic":"Coding with Claude"}]},{"topic":"Tuesday","children":[{"topic":"Evening Cooking Class"}]},{"topic":"Wednesday","children":[{"topic":"Lunch with Coworkers"}]},{"topic":"Thursday","children":[{"topic":"Night Book Club Meeting"}]},{"topic":"Friday","children":[{"topic":"Afternoon Therapy Appointment"}]},{"topic":"Saturday","children":[{"topic":"Morning Farmers Market Trip"}]},{"topic":"Sunday","children":[{"topic":"Family Dinner"}]},{"topic":"Daily","children":[{"topic":"30-minute Meditation"}]},{"topic":"Bi-Weekly","children":[{"topic":"Date Night with Partner"}]},{"topic":"Monthly","children":[{"topic":"Budget Review"}]},{"topic":"Quarterly","children":[{"topic":"Dentist Check-up"}]},{"topic":"Yearly","children":[{"topic":"Vacation Planning"}]}]}
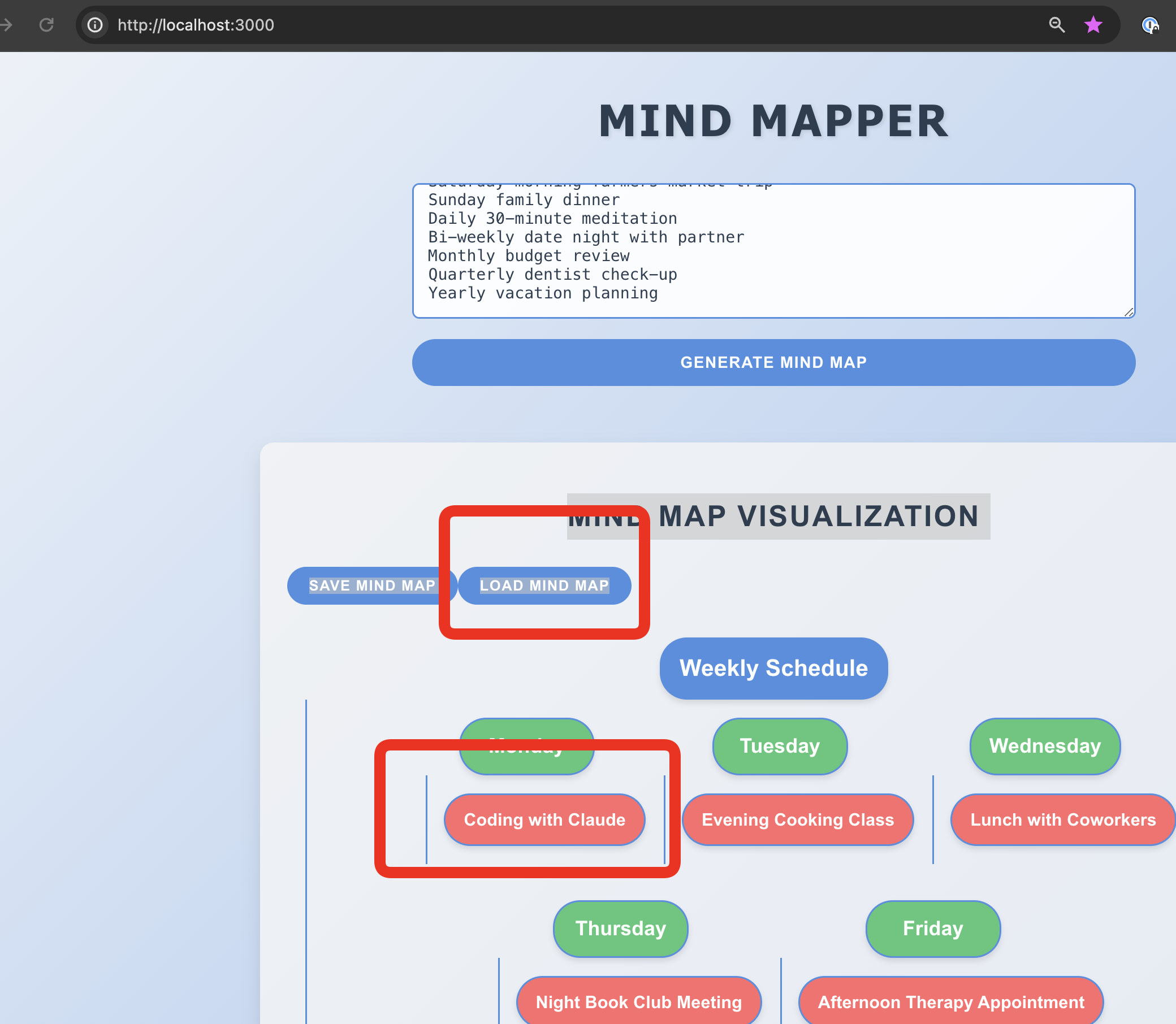
As you can see, after editing and loading the modified mind map, it works.
(Optional) Incrementally add a "Thought!" to the mind map
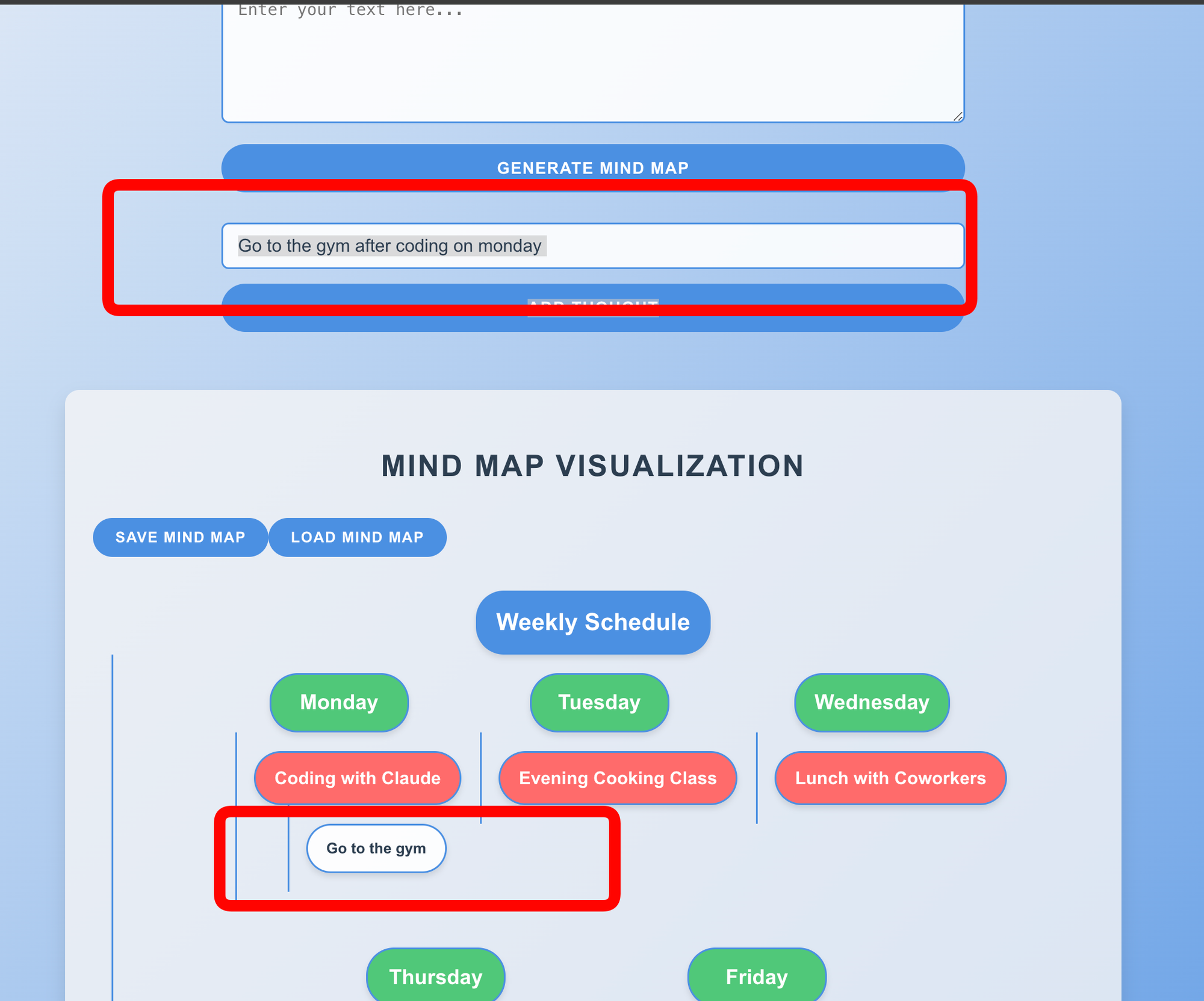
Conclusion
This shows how you can keep the architecture documentation in sync with the code, and keep an eye on implementing coherent code that new developers or architects can easily understand before digging deeper into the code, making it easy to maintain.
Here are a few more tips on this and code generation in general:
- Be sure to check the LLM models (e.g. gpt-4, Sonnet, etc.) used; sometimes Claude replaces models without “telling” you.
- Make sure you commit your code after each feature/bug fix, so you can easily roll back to a previous version, although Claude will ask you before saving anyway.
- Try to keep your code as modular as possible; monolithic files with too many functions are difficult to edit due to Claude’s (and other LLMs’) laziness syndrome.
- Make sure you test thoroughly, as bulk changes can break old working code and roll back (check out the previous git version to undo).
- Although Claude has become relatively cheap, keep an eye on the costs; the more you generate (especially reading files), the more it is not free. But I think for most of us the time saved justifies the cost by a factor of X.
- Claude sometimes tries to rationalise things that he thinks can be safely removed. To be on the safe side, add “Don’t change anything else” at the end of your prompts.
Comments ()