A Comprehensive Guide to Fine-Tuning Techniques for LLMs
This article explores fine-tuning techniques for Large Language Models, detailing methods available in the Hugging Face TRL library to enhance model performance for specific tasks.
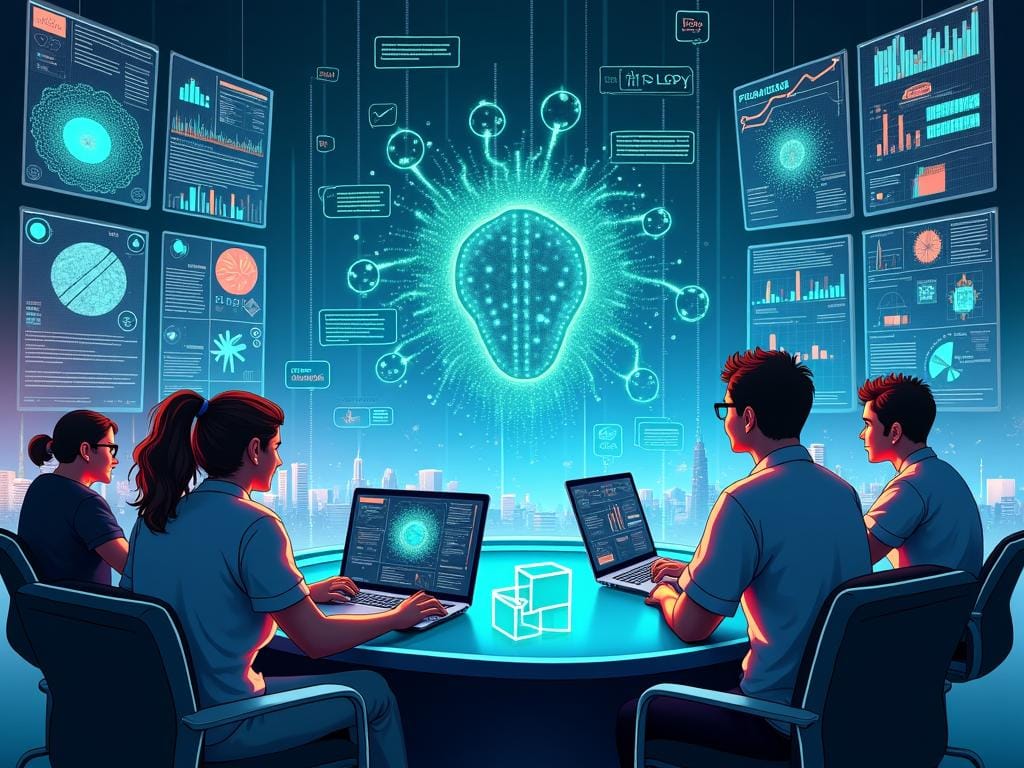
This article explores fine-tuning techniques for Large Language Models, detailing methods available in the Hugging Face TRL library to enhance model performance for specific tasks.